Introduction
Search functionality is one of the most critical aspects of any website, especially for eCommerce stores. If customers can't find what they're looking for, they won't make a purchase. WordPress and WooCommerce offer built-in search features, but they have several limitations that can frustrate users.
In this article, we'll explore how WordPress and WooCommerce search works, their limitations, and how you can enhance search functionality to deliver better results. We'll also introduce Advanced Woo Search, a powerful plugin that makes improving your store's search effortless.
How WordPress Search Works
By default, WordPress uses a simple search mechanism based on the database's wp_posts
table. Here's how it works:
- WordPress searches for the entered keyword in the post title, post content, and excerpt.
- It uses the LIKE query in MySQL, which is not optimized for performance on large databases.
- It does not search in custom fields, taxonomies, product SKUs, or other metadata.
- Results are typically sorted by date rather than relevance.
As seen, it's simply search inside several most common post fields (title, content, excerpt) and performs SQL query LIKE '%s%'. So if at least one search word is found inside any post content in any position (word beginning, middle, or end), it will be included in the search results.
Limitations of Default WordPress Search
- Doesn't support searching within custom fields, SKUs, taxonomies, etc.
- No option to exclude certain products from search results based on taxonomies, meta field, etc.
- No support for fuzzy matching, meaning slight typos lead to no results.
- Search can only work as partial match following schema %s%. No option to use whole match search only.
- No option for live search results by default.
- Performance issues on large websites with thousands of posts/products.
As seen, default WordPress search is quite limited and provides only a very basic search mechanism.
What About WooCommerce Search?
WooCommerce slightly extends WordPress search by including products and their descriptions, but it still relies on the same basic search mechanism. The key differences include:
- WooCommerce ensures that product pages appear in search results.
- It searches within product titles and descriptions but not in SKUs or custom fields.
- No built-in support for filtering results by category, tags, or other product attributes.
- Performance can be slow on large stores with many products.
So the main difference here is that products appear inside search results, but search mechanics overall stay the same with the same limitations.
Conclusion: What WordPress/WooCommerce Can and Can't Do
Let's sum up what features WordPress/WooCommerce default search has and doesn't have.
Feature | Default WordPress Search | Default WooCommerce Search |
---|---|---|
Search in titles | ✅ | ✅ |
Search in content/descriptions | ✅ | ✅ |
Search in custom fields | ❌ | ❌ |
Search by SKU | ❌ | ❌ |
Search in product attributes | ❌ | ❌ |
Filtering search results | ❌ | ❌ |
Search in categories and tags | ❌ | ❌ |
Relevance-based sorting | ❌ | ❌ |
Performance on large stores | 🚫 Slows down | 🚫 Slows down |
How to Extend WordPress and WooCommerce Search
If you want to improve your search functionality, you have two main options: manually modifying the search or using a specialized plugin.
Adding Advanced Search Features Manually
- Search by SKU: Modify the WooCommerce product query to include SKU searches in
meta_query
. - Search custom fields: Use
WP_Query
withmeta_query
to include custom field values in search results. - Improve relevance sorting: Use a custom SQL query or custom plugins to prioritize matches.
- Enable taxonomy-based filtering: Modify queries to include product categories, tags, and attributes.
Below we will cover some ways to manually extend default WordPress search.
Adding SKUs search
Let's now cover how we can enable SKUs search for default WooCommerce search mechanics.
We can do this by simply using the following code snippet.
function custom_search_by_sku( $search, $wp_query ) { global $wpdb; if ( is_admin() || !$wp_query->is_search() ) { return $search; } $search_query = get_search_query(); if ( empty( $search_query ) ) { return $search; } // Search in post title, content, and SKU meta field $search = " AND ( ({$wpdb->posts}.post_title LIKE '%" . esc_sql( $search_query ) . "%') OR ({$wpdb->posts}.post_content LIKE '%" . esc_sql( $search_query ) . "%') OR ({$wpdb->posts}.post_excerpt LIKE '%" . esc_sql( $search_query ) . "%') OR EXISTS ( SELECT 1 FROM {$wpdb->postmeta} WHERE {$wpdb->postmeta}.post_id = {$wpdb->posts}.ID AND {$wpdb->postmeta}.meta_key = '_sku' AND {$wpdb->postmeta}.meta_value LIKE '%" . esc_sql( $search_query ) . "%' ) ) "; return $search; } add_filter( 'posts_search', 'custom_search_by_sku', 10, 2 );
Adding taxonomies search
To enable search by category taxonomy for posts and product_category taxonomy for WooCommerce products we can use the following code snippet.
function custom_search_by_category( $search, $wp_query ) { global $wpdb; if ( is_admin() || !$wp_query->is_search() ) { return $search; } $search_query = get_search_query(); if ( empty( $search_query ) ) { return $search; } // Search in post title, content, SKU meta field, and product categories $search = " AND ( ({$wpdb->posts}.post_title LIKE '%" . esc_sql( $search_query ) . "%') OR ({$wpdb->posts}.post_content LIKE '%" . esc_sql( $search_query ) . "%') OR EXISTS ( SELECT 1 FROM {$wpdb->term_relationships} tr INNER JOIN {$wpdb->term_taxonomy} tt ON tr.term_taxonomy_id = tt.term_taxonomy_id INNER JOIN {$wpdb->terms} t ON tt.term_id = t.term_id WHERE tr.object_id = {$wpdb->posts}.ID AND tt.taxonomy IN ('category', 'product_cat') AND t.name LIKE '%" . esc_sql( $search_query ) . "%' ) ) "; return $search; } add_filter( 'posts_search', 'custom_search_by_category', 10, 2 );
Adding custom fields search
Lastly, let's find how to enable search by a specific custom field. In our example, we will enable search by my_custom_field
field, but you can change it to any other.
So to enable search by my_custom_field
custom field, please use the following code snippet.
function custom_search_by_sku_category_and_custom_field( $search, $wp_query ) { global $wpdb; $field_name = 'my_custom_field'; if ( is_admin() || !$wp_query->is_search() ) { return $search; } $search_query = get_search_query(); if ( empty( $search_query ) ) { return $search; } // Search in post title, content, SKU, custom field, and categories $search = " AND ( ({$wpdb->posts}.post_title LIKE '%" . esc_sql( $search_query ) . "%') OR ({$wpdb->posts}.post_content LIKE '%" . esc_sql( $search_query ) . "%') OR EXISTS ( SELECT 1 FROM {$wpdb->postmeta} WHERE {$wpdb->postmeta}.post_id = {$wpdb->posts}.ID AND {$wpdb->postmeta}.meta_key = '{$field_name}' AND {$wpdb->postmeta}.meta_value LIKE '%" . esc_sql( $search_query ) . "%' ) ) "; return $search; } add_filter( 'posts_search', 'custom_search_by_sku_category_and_custom_field', 10, 2 );
Conclusion
As seen, everything is possible and you can manually extend default WordPress search to work with taxonomies, SKUs, custom fields, and many more, but it can take a significant amount of time and coding skills.
Also, such a solution is limited: what if you want to search for several custom fields? What if you want to have an option to change that field from the admin dashboard? What about search filtering?
These are only a few questions that can come to mind along with many others.
Good news is that you can add all these features (and many others) with a hassle-free way. We will cover this way below.
The Easy Way: Using Advanced Woo Search
If you're looking for a hassle-free way to enhance WooCommerce search, Advanced Woo Search is the perfect solution. It's a powerful plugin that adds all the missing search capabilities without requiring custom coding.
Why Choose Advanced Woo Search?
- ✅ Search by SKUs – Find products by SKU instantly.
- ✅ Search by custom fields – Includes product attributes, meta fields, and more.
- ✅ Search by taxonomies – Choose only needed taxonomies to search in.
- ✅ Supports categories and tags display – Search for taxonomies archive pages.
- ✅ Filtering – Filter products based on various parameters.
- ✅ Relevance-based sorting – Displays the most relevant results first.
- ✅ Fast and optimized – Works efficiently even on large WooCommerce stores.
- ✅ Live search – Search and display product as you type.
- ✅ Easy setup – No coding required, just install and configure.
How to Set Up Advanced Woo Search
- 1. Install the Plugin – Download from WordPress plugin repository (free version) or email (paid version) and install it.
- 2. Start indexing products content - this needs to be done only one time. Products index is needed to create a fast and reliable search experience.
- 3. Configure Search Settings – Choose which fields to include (title, SKU, custom fields, categories, etc.).
- 4. Customize the Appearance – Adjust the look and feel of search results.
- 5. Test the Search – Ensure everything works as expected.
How to enable search sources
Let's dive a bit more into how you can enable different search sources and what sources are available at all.
So after you enable and activate Advanced Woo Search plugin - proceed to plugin settings page -> Performance tab. Then scroll down to Data to Index option.
Here you can find all possible fields that it is possible to search in. Before making it possible for searching, we need to enable needed ones and index its content.
So, for example, we need to enable SKU and attributes field. For attributes, we want to make only Brand attribute content available for searching.
1. First enable SKU and Attributes fields.
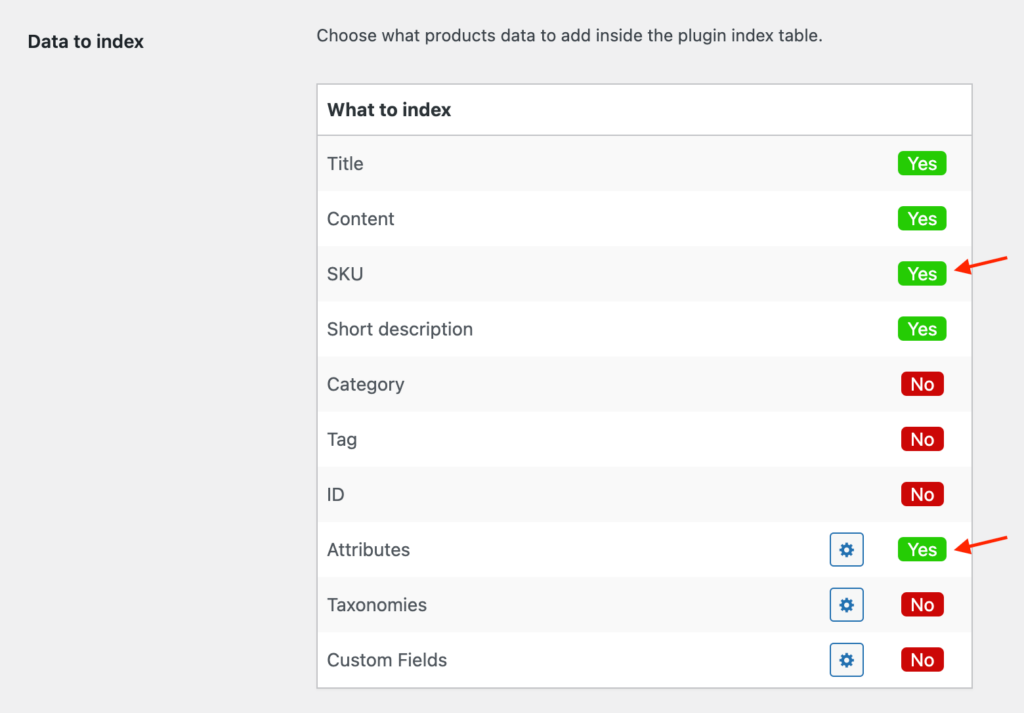
Enable product field for indexing
2. Then click on gear icon near Attributes field.

Attributes field settings
3. On the next page enable Brand attribute.
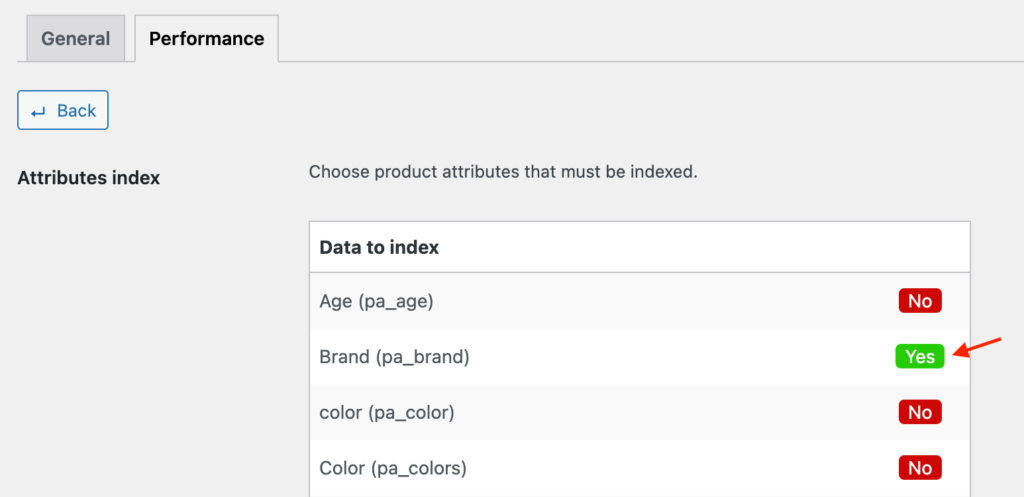
Brand attribute
4. Lastly go to the main plugin settings page and Reindex table.

Index button
We enable and indexed needed fields, but it is not all. Now we need to enable that field for searching.
To do that go to the Search Results settings tab and find Search In option.
For this option simply enable the same field and don't forget about Brand attribute inside Attributes settings.
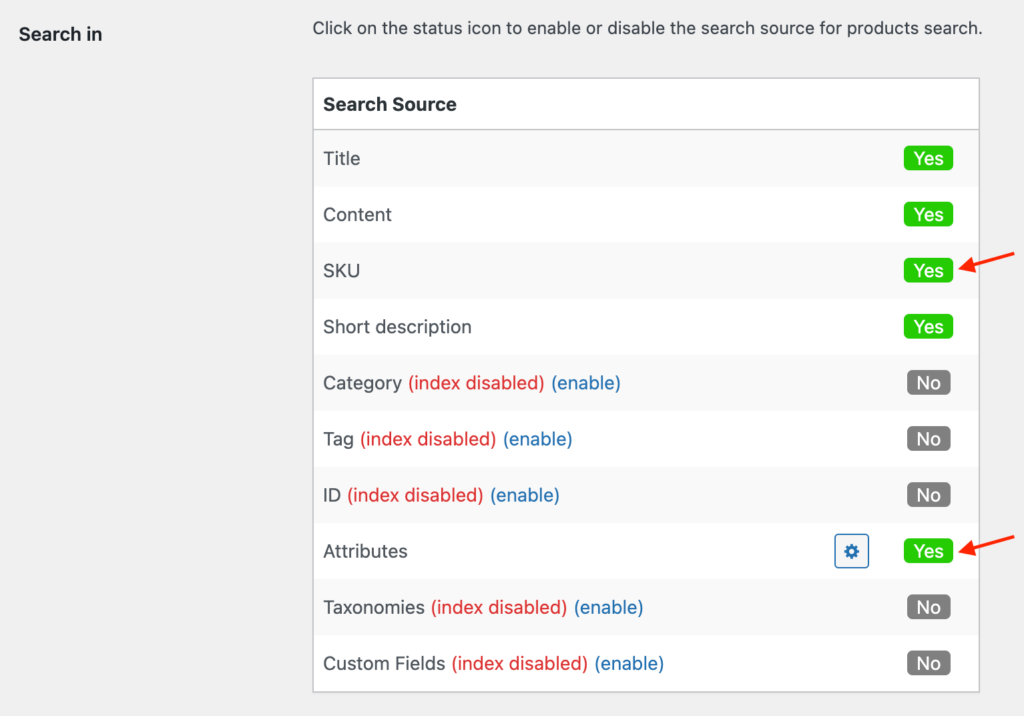
Search In option field
That's all! We enable SKU and Attributes search without any custom code snippets - just by using simple plugin settings with a large amount of variation.
How to create search results filters
Let's cover how to create a search filter for products search results.
As was mentioned - it is a feature that default WooCommerce search is lacking, but with Advanced Woo Search plugin you can simply create any type of such filter.
For example - let's create a filter based on product category and hide all products with ABC category from the search results.
1. Open plugin settings page -> Search Results tab and scroll to Filter Results section.
2. Click Filter products search results button to create a new search results filter.
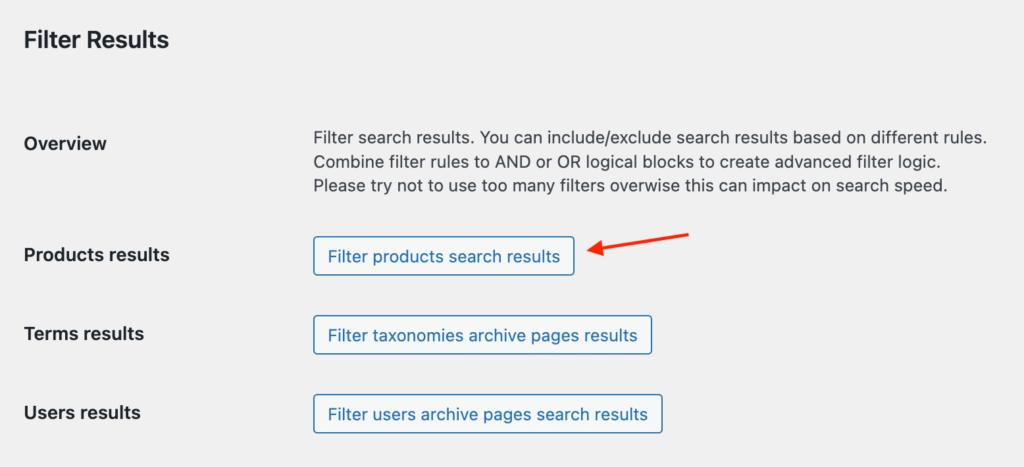
Search filters button
3. Now set following parameters for your filtering rule:
Product category -> not equal to -> ABC

Search filter based on product category
4. Lastly just save changes and check your search results - all products with ABC category must be excluded.
Conclusion
The default WordPress and WooCommerce search features are basic and have significant limitations. If you want to provide a better search experience for your customers, you need to extend the default functionality.
While you can manually modify the search system, the easiest and most effective solution is using Advanced Woo Search. It enhances WooCommerce search with SKU support, custom field searches, relevance-based sorting, and more—all without requiring any coding.
Ready to upgrade your store's search? Get Advanced Woo Search today!
Trackbacks